函数指针是 C 语言中一项非常重要的特性,对于 C/C++ 程序员是必须要掌握的技术。函数指针提供了一种灵活的方式来操作函数,允许在运行时动态地选择要执行的函数。
1. 函数名理解
函数名可以被视为指向函数的指针。当你使用函数名时,它会自动转换为函数的指针,指向函数的入口地址。即:我们可以通过指针间接调用函数。请看下面示例代码:
#include <stdio.h> void demo() { printf("hello demo\n"); } int main() { // 1. 直接调用函数 demo(); // 2. 函数名为指向函数入口地址的指针 printf("%ld\n", demo); // 3. 间接调用函数 void(*func)() = demo; (*func)(); return 0; }
程序运行结果:
hello demo 4363710224 hello demo
2. 函数指针类型
函数指针类型如何定义?请看下面示例代码:
#include <stdio.h> int demo(int a, int b) { return a + b; } int main() { // 1. 第一种方式 int(*func1)(int, int) = demo; int result = func1(10, 20); printf("result = %d\n", result); // 2. 第二种方式 typedef int(FUNC_TYPE)(int, int); FUNC_TYPE* func2 = demo; result = func2(10, 20); printf("result = %d\n", result); // 3. 第三种方式 typedef int(FUNC_POINTER)(int, int); FUNC_POINTER* func3 = demo; result = func3(10, 20); printf("result = %d\n", result); return 0; }
程序运行结果:
result = 30 result = 30 result = 30
3. 函数指针数组
函数指针数组顾名思义就是数组中的所有元素都是函数指针类型。函数指针数组提供了一种动态选择和调用函数的机制,适用于需要根据不同的条件选择不同的函数执行的场景。它们在命令调度、事件处理、状态机、算法实现和插件化架构等方面都有广泛的应用。
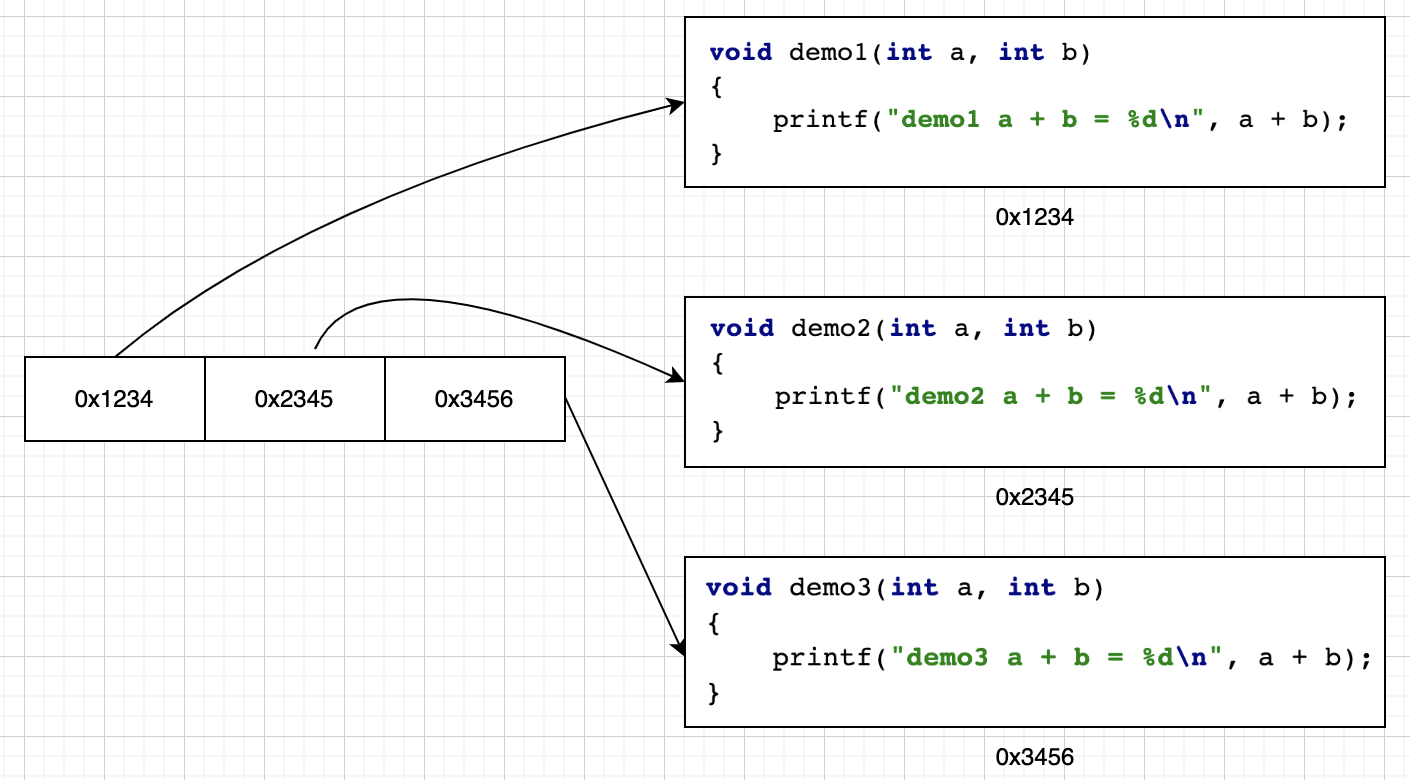
#include <stdio.h> void demo1(int a, int b) { printf("demo1 a + b = %d\n", a + b); } void demo2(int a, int b) { printf("demo2 a + b = %d\n", a + b); } void demo3(int a, int b) { printf("demo3 a + b = %d\n", a + b); } int main() { void(*func_array[])(int, int) = {demo1, demo2, demo3}; // 调用数组中的函数 func_array[1](10, 20); func_array[0](30, 40); func_array[2](50, 60); return 0; }
程序运行结果:
demo2 a + b = 30 demo1 a + b = 70 demo3 a + b = 110
4. 函数指针作回调函数
回调函数是一种编程模式,它允许将一个函数的指针作为参数传递给另一个函数,并在特定事件或条件发生时,通过调用该函数指针来回调执行相应的操作。回调函数是一种灵活的方式,用于实现事件驱动和异步编程。
在回调函数的模式中,存在两个主要角色:
- 调用方:调用方是一个函数或模块,它需要执行某些操作,并在适当的时候调用回调函数。
- 回调函数:回调函数是由调用方提供的一个函数,它定义了在特定事件发生时应该执行的操作。回调函数的指针被传递给调用方,在合适的时机由调用方调用。
示例代码:
#include <stdio.h> int do_logic(int(*func)(int, int)) { int a = 20; int b = 10; int result = func(a, b); return result; } int my_func1(int a, int b) { return a + b; } int my_func2(int a, int b) { return a - b; } int main() { // 函数名做函数参数 int result = do_logic(my_func1); printf("result = %d\n", result); result = do_logic(my_func2); printf("result = %d\n", result); return 0; }
程序执行结果:
result = 30 result = 10
5. 函数指针作函数返回值
函数指针可以作为函数的返回值,这在一些情况下非常有用。例如,可以编写一个函数,根据输入的参数返回不同的函数指针,这样可以根据不同的条件选择不同的函数执行,实现更加动态的程序逻辑。
#include <stdio.h> #include <string.h> int my_func1(int a, int b) { return a + b; } int my_func2(int a, int b) { return a - b; } typedef int(*FUNC_POINTER)(int, int); FUNC_POINTER do_logic(const char *type) { if (0 == strcmp(type, "plus")) { return my_func1; } else if(0 == strcmp(type, "minus")) { return my_func2; } return NULL; } int main() { // 函数名做函数参数 int result = do_logic("plus")(10, 20); printf("result = %d\n", result); result = do_logic("minus")(10, 20); printf("result = %d\n", result); return 0; }
程序运行结果:
result = 30 result = -10