多项式回归时线性回归模型的一种,使用多项式可以逼近任意函数,因此多项式回归有着广泛的应用。
多项式回归的最大优点就是可以通过增加 x 的高次项对样本集进行逼近,直至达到目标为止。在通常的实际问题中,不论依变量与其他自变量的关系如何,我们总可以用多项式回归来进行分析。
在 scikit-learn 中,使用多项式回归的步骤如下:
- 根据已有的特征构建多项式特征
- 使用 LinearRegression 拟合多项式特征数据
下面是一个多项式回归的代码例子:
import numpy as np import sympy import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression from sklearn.preprocessing import PolynomialFeatures def poly_regression(degree): """degree 为最高此项""" x = [-10., -7.78, -5.56, -3.33, -1.11, 1.11, 3.33, 5.56, 7.78, 10.] y = [107., 69.49, 31.86, 11.11, -0.77, 4.24, 20.11, 29.86, 59.49, 95.] # 绘制散点图 plt.scatter(x, y) # 将列表 x、y 转换为矩阵,并转置 x = np.mat(x).transpose() y = np.mat(y).transpose() # 构建多项式特征, degree 指定最高此项 x = PolynomialFeatures(degree=degree, include_bias=False).fit_transform(x) # 线性回归对象 linear_model = LinearRegression() linear_model.fit(x, y) # 构建多项式方程 x = sympy.Symbol("x") items = [] ratio_list = linear_model.coef_[0] for i, w in enumerate(ratio_list): items.append(w * x ** (i + 1)) y = sum(items) + linear_model.intercept_[0] x = np.linspace(-10, 10, 10) y = [y.subs({"x": v}) for v in x] # 绘制折线 plt.plot(x, y) plt.show() if __name__ == "__main__": poly_regression(1) poly_regression(3) poly_regression(5) poly_regression(8)
程序执行结果为:
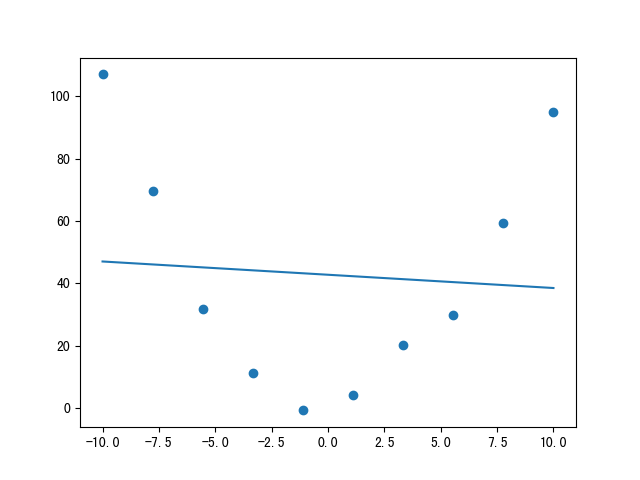
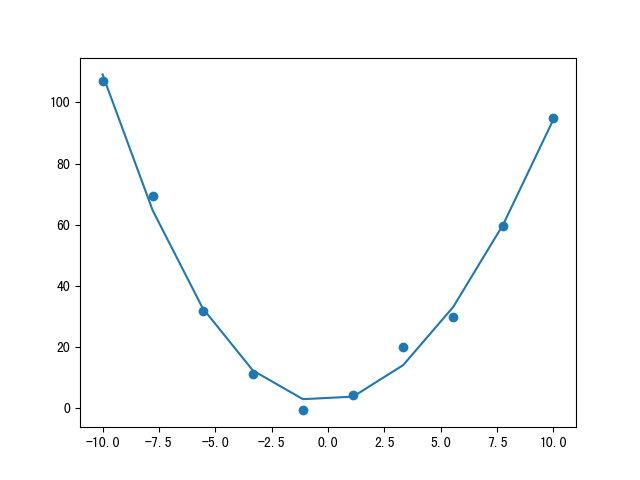
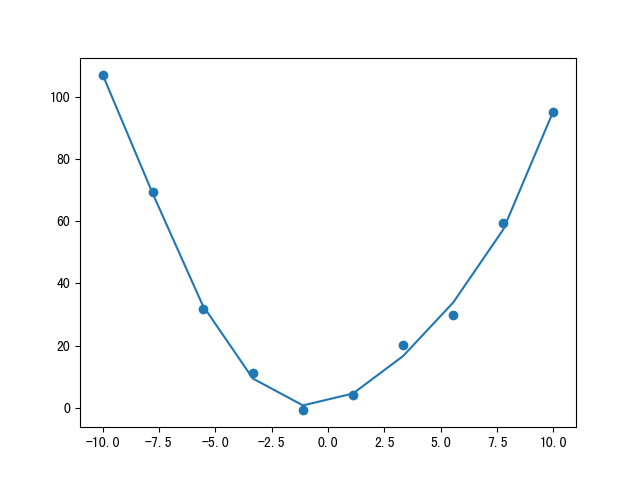
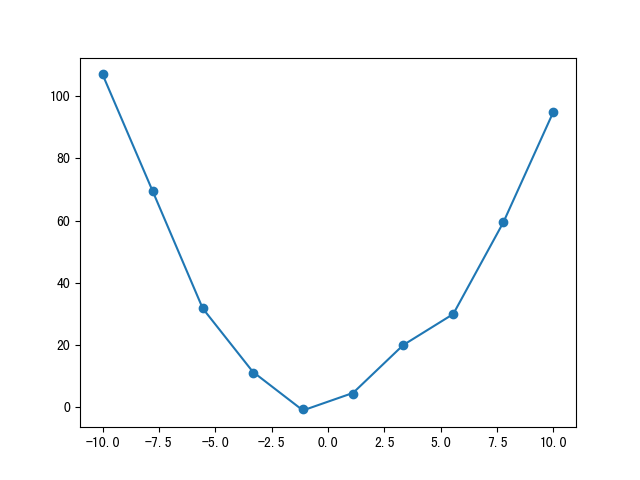
随着 degree 的变大,拟合效果越来越好。但是,并不是最高此项越高,拟合效果就一定越好。